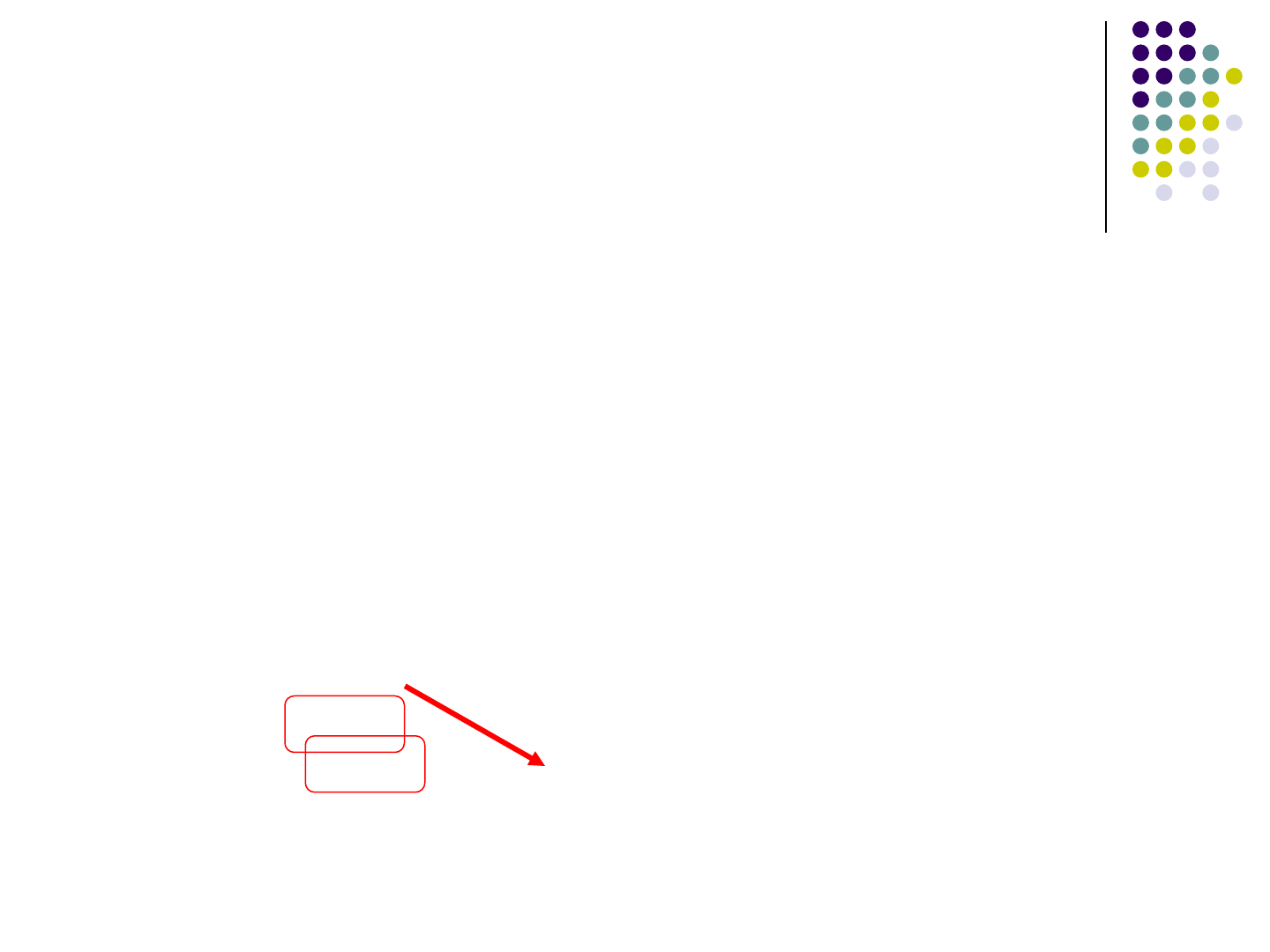
User-Visible Constant Strings in
Web Applications
Constant Strings outside Tags
echo "and pressed 'refresh' on your browser.
In this case, your responses have<br/>\n";
echo "already been saved."
echo "</font></center><br /><br />";
(from question.php, Lime Survey 0.97)
Constant Strings in value attribute of input tags
if (substr(strtolower($reply_subj), 0, 3) != "re:")
$reply_subj = "Re: ".$reply_subj;
echo " <INPUT TYPE=TEXT NAME=passed_subject
SIZE=60 VALUE=\"$reply_subj\">";
(from compose.php, SquirrelMail 0.2.1)
106