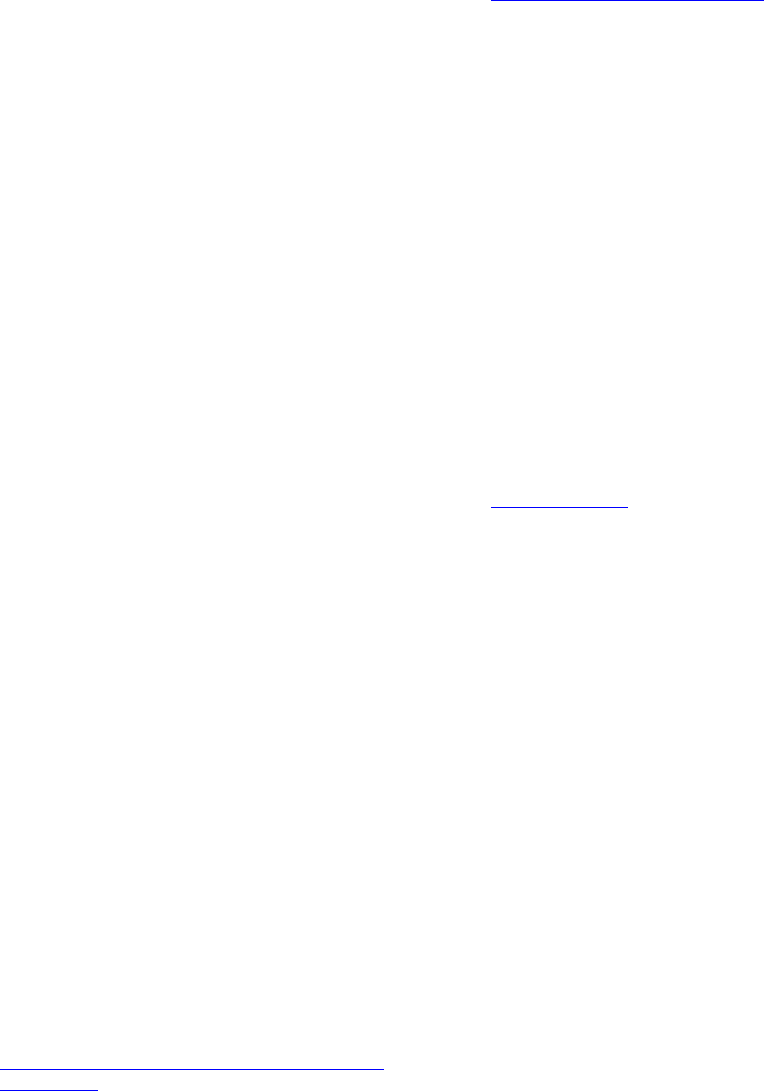
5. Conclusion and future work
In this paper, we presented an automated fix
generation solution for replacing vulnerable
Statement-based SQL statements with secured
PreparedStatement-based SQL statements. We
use a conversion algorithm where we secure a
statement without knowledge of the context while
maintaining the logic of the statement. The intuition
behind this solution is that automated fix generation
can be a means for securing vulnerable SQL
statements without requiring expertise in software
security. In addition, since our solution replaces the
vulnerable statement with a secured prepared
statement, then our solution removes the SQLIVs and
is not prone new types of SQLIAs. Further, our
solution is not disadvantaged by the same
computational overhead that dynamic statement
analysis solutions generate since our solution pre-
compiles the SQL statement.
In our future work, we will develop our solution
into an Eclipse IDE plug-in that we will use to conduct
further tests of our solution. In addition, we are going
to refine our conversion algorithm to reduce the cases
of vulnerable SQL statements that it cannot handle to a
minimal set. We are looking for corporate partners
that we could work with to test our solution.
We would also like to note that while this work is
initially for Java, the concept and general algorithm
could be applied to other languages that meet the
environment assumptions as well. We surmise that for
any positive results that are gained through this
solution in Java, similar results could be gained in
other languages.
6. References
[1] S. Barnum, McGraw, G., "Knowledge for Software
Security," Security & Privacy Magazine, IEEE, vol.
3, no. 2, 2005, pp. 74 - 78.
[2] G. Buehrer, B. W. Weide, and P. A. G. Sivilotti,
"Using Parse Tree Validation to Prevent SQL
Injection Attacks," 5th International Workshop on
Software Engineering and Middleware, Lisbon,
Portugal, 2005, pp. 106-113.
[3] Eclipsepedia, What is a Quick Fix?, 2006,
http://wiki.eclipse.org/index.php/FAQ_What_is_a_Q
uick_Fix%3F, accessed January 16, 2007.
[4] D. Florescu, C. Hillery, D. Kossmann, P. Lucas, F.
Riccardi, T. Westmann, J. Carey, and A.
Sundararajan, "The BEA Streaming XQuery
Processor," The VLDB Journal, vol. 13, no. 3, 2004,
pp. 294-315.
[5] P.-Y. Gibello, Zql: A java sql parser, 2006,
http://www.experlog.com/gibello/zql/
, accessed
January 16, 2007.
[6] N. Gupta, A. P. Mathur, and M. L. Soffa,
"Automated Test Data Generation Using an Iterative
Relaxation Method," 6th ACM SIGSOFT
International Symposium on Foundations of Software
Engineering, Lake Buena Vista, Florida, United
States, 1998, pp. 231-244.
[7] W. G. J. Halfond and A. Orso, "AMNESIA: Analysis
and Monitoring for NEutralizing SQL-Injection
Attacks," 20th IEEE/ACM International Conference
on Automated Software Engineering, Long Beach,
CA, USA, 2005, pp. 174-183.
[8] W. G. J. Halfond and A. Orso, "Combining Static
Analysis and Runtime Monitoring to Counter SQL-
Injection Attacks," 3rd International Workshop on
Dynamic Analysis, St. Louis, Missouri, 2005, pp. 1-
7.
[9] D. Hovemeyer and W. Pugh, "Finding Bugs is Easy,"
19th Annual ACM SIGPLAN Conference on Object-
Oriented Programming Systems, Languages, and
Applications, Vancouver, BC, CANADA, 2004, pp.
92-106.
[10] NIST, National Vulnerability Database, 2007,
http://nvd.nist.gov/
, accessed January 16, 2007.
[11] R. Ramler and K. Wolfmaier, "Economic
Perspectives in Test Automation: Balancing
Automated and Manual Testing with Opportunity
Cost," 2006 International Workshop on Automation
of Software Test, Shanghai, China, 2006, pp. 85-91.
[12] Z. Su and G. Wassermann, "The Essence of
Command Injection Attacks in Web Applications,"
33rd ACM SIGPLAN-SIGACT Symposium on
Principles of Programming Languages, Charleston,
South Carolina, USA, 2006, pp. 372-382.