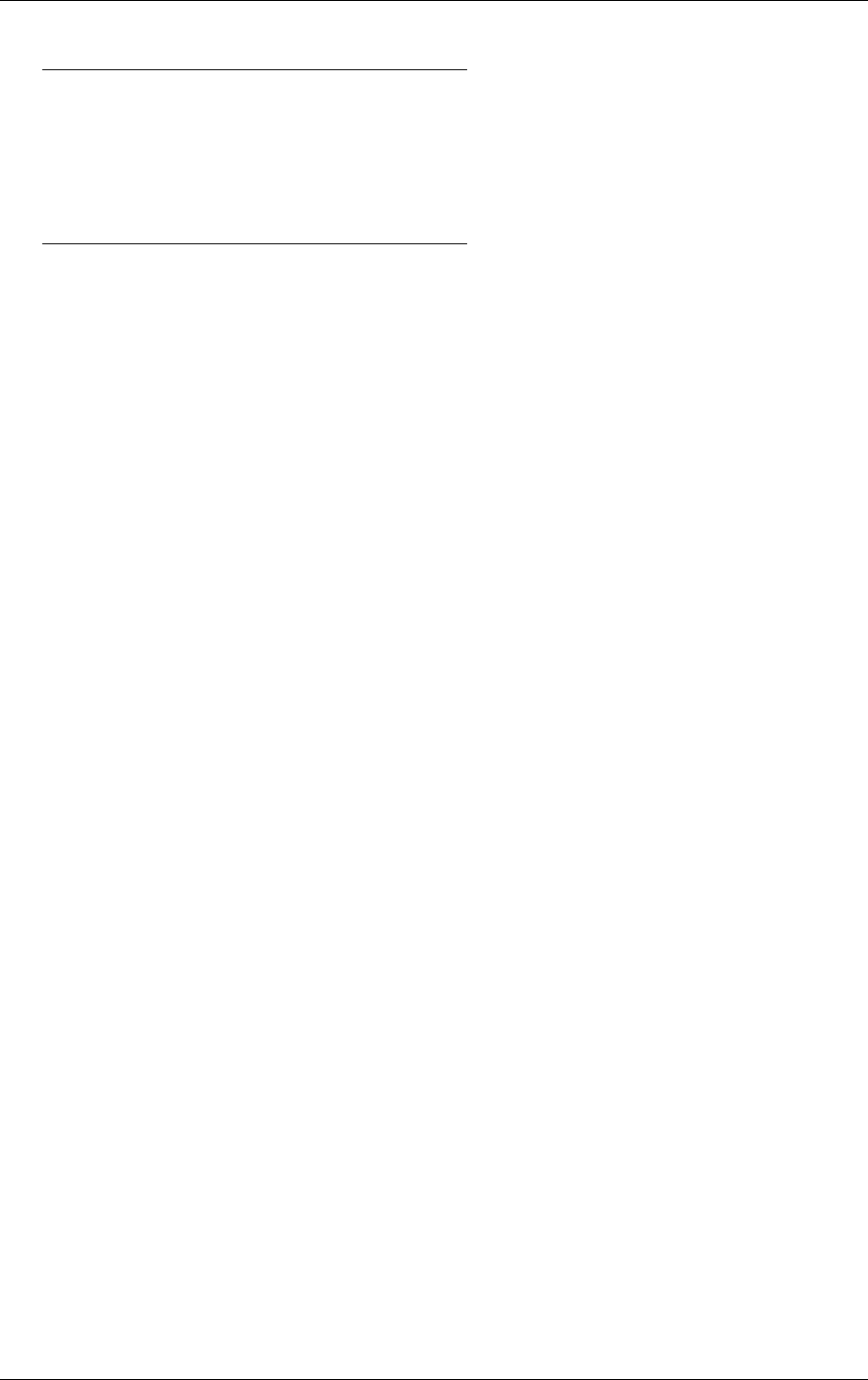
5.3 Sphere build inputs 5 Acceleration structures
Curve type Control points per segment
Piecewise linear 2
Quadratic 3
Cubic 4
Catmull-Rom 4
Bézier 4
NVIDIA OptiX considers each polynomial segment to be a primitive, with its own primitive
ID.
A curve build input (OptixBuildInputCurveArray) references an array of vertex buffers in
device memory, one buffer per motion key (a single vertex buffer if there is no motion). (See
“Motion blur” (page 34).) Parallel to this, there is an array of radius buffers in device memory,
one buffer per motion key, providing a radius value at each control vertex at each motion key.
There is also a (required) index buffer in device memory. Ribbons can also reference optional
normal buffers that are parallel to the vertex buffers.
The B-spline control points of each curve strand will appear sequentially in the vertex buffer.
The index array contains one index per segment, namely, the index of the segment’s first
control point. For example, a cubic curve with three segments will have six vertices. The
index array might contain {10, 11, 12}, in which case the 3 segments will have control
points: {v[10], v[11], v[12], v[13]}, {v[11], v[12], v[13], v[14]} and
{v[12], v[13], v[14], v[15]}.
The vertex buffers for ribbons store quadratic B-spline control points whereas the normal
buffers contain the normals at borders of the ribbon segments. A ribbon strand with three
segments will store five control points. If normals are specified, four normals will be required
for three segments. They are linearly interpolated along the curve segments. For example, the
ribbon strand might have indices {0, 1, 2}. In this case, the control points of the segments
would be {v[0], v[1], v[2]}, {v[1], v[2], v[3]} and {v[2], v[3], v[4]}, the
normals {n[0], n[1]}, {n[1], n[2]} and {n[2], n[3]}. The segment with index i will
use control points {v[i], v[i+1], v[i+2]} and normals {n[i], n[i+1]}. Note that there
is one more control point than normals in the ribbon strand. Since the indices are used for
addressing both vertices and normals, the normals need to be padded with an unused vector
at the end of the strand.
End caps appear at the ends of strands. NVIDIA OptiX detects the strands by checking the
overlap of segment control points. Within a B-spline strand, adjacent segments overlap all but
one of their control points. In other words, unless indexArray[N+1] is equal to
indexArray[N]+1, segment N is the end of one strand and segment N+1 is the beginning of
another.
See also
“Differences between curves, spheres, and triangles” (page 85) .
5.3 Sphere build inputs
Similar to curves, NVIDIA OptiX supports spheres as geometric primitives. Spheres can be
used in different applications to represent, for example, molecules, spray, or smoke.
Each sphere is defined by a three-dimensional center point and a radius.
© 2023 NVIDIA Corporation NVIDIA OptiX 7.7 ± Programming Guide 25