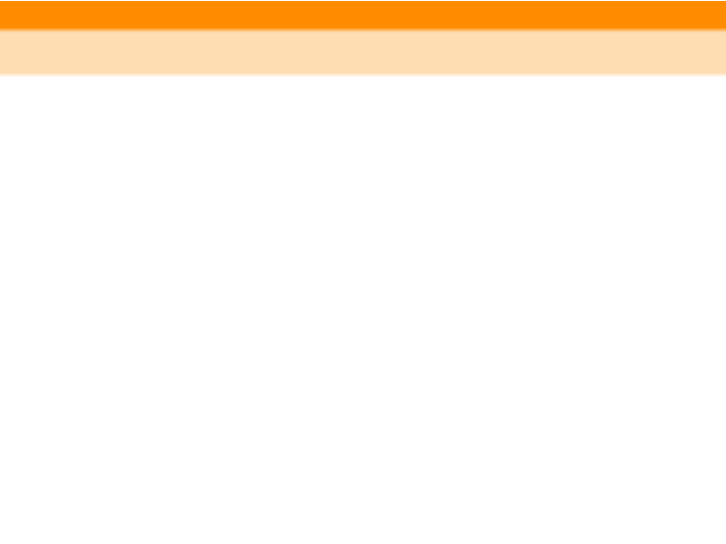
Testing strategies Examples
Automation of binary search test, version 5
Output (buggy version):
FF
======================================================================
FAIL: test
_
all (
__
main
__
.TestBinarySearch)
Search for all items in an array
----------------------------------------------------------------------
Traceback (most recent call last):
File "src/binsearch.py", line 238, in test
_
all
self.check
_
item(x, x[i], i)
File "src/binsearch.py", line 231, in check
_
item
self.assertEqual(result, expected, msg)
AssertionError: binary
_
search(x, 0) = -1, expected 0
x = [0]
======================================================================
FAIL: test
_
equal
_
elems (
__
main
__
.TestBinarySearch)
Search an array whose elements are all equal
----------------------------------------------------------------------
Traceback (most recent call last):
File "src/binsearch.py", line 253, in test
_
equal
_
elems
self.assert
_
(result in range(0,size), msg)
AssertionError: binary
_
search(x, 10) = -1, expected [0,1)
x = [10]
----------------------------------------------------------------------
Ran 2 tests in 0.001s
FAILED (failures=2)