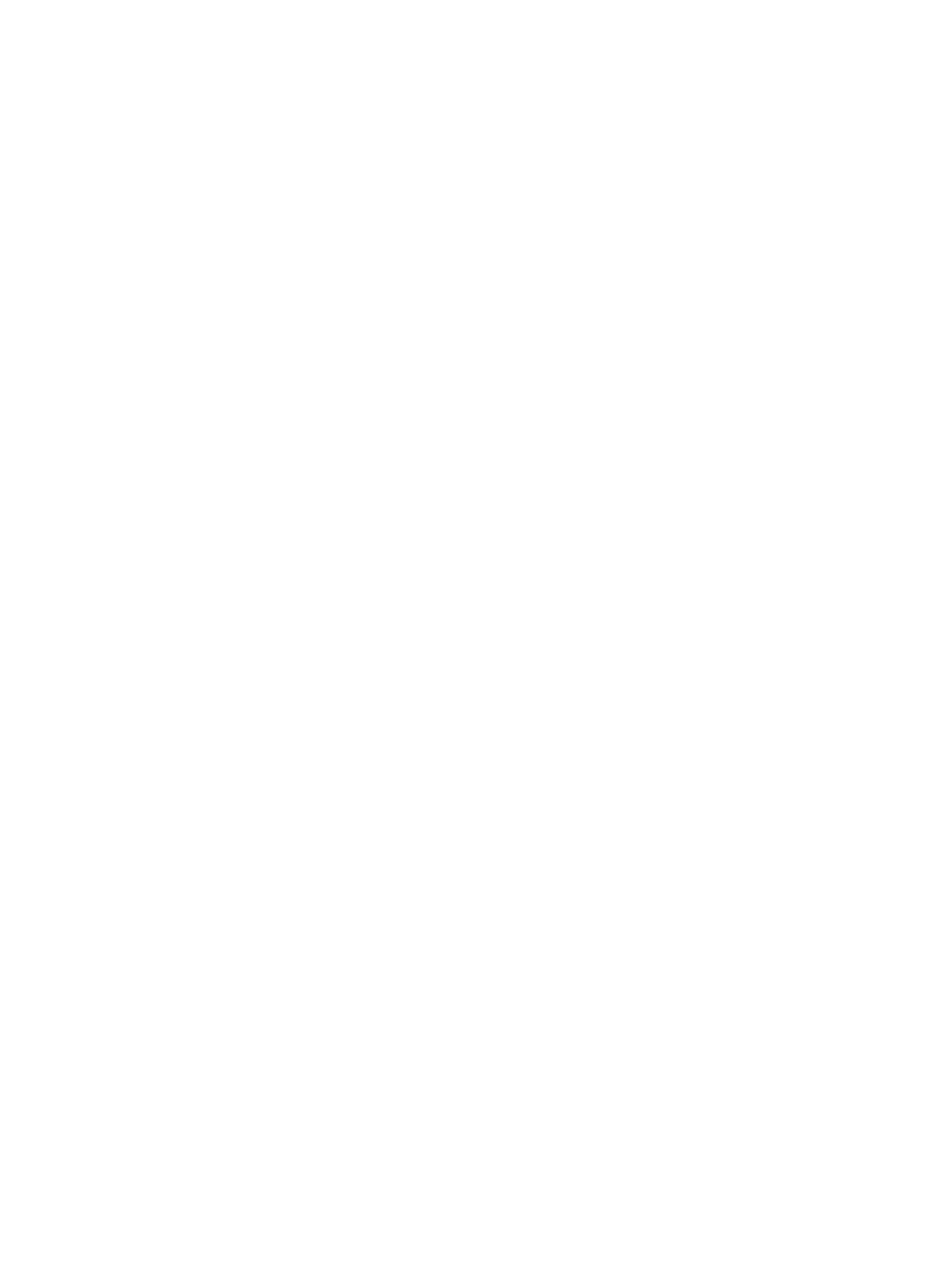
Queries in LINQ to DataSet
A query is an expression that retrieves data from a data source. Queries are usually expressed in a specialized query
language, such as SQL for relational databases and XQuery for XML. Therefore, developers have had to learn a new query
language for each type of data source or data format that they query. Language-Integrated Query (LINQ) offers a simpler,
consistent model for working with data across various kinds of data sources and formats. In a LINQ query, you always work
with programming objects.
A LINQ query operation consists of three actions: obtain the data source or sources, create the query, and execute the query.
Data sources that implement the IEnumerable(Of T) generic interface can be queried through LINQ. Calling AsEnumerable
on a DataTable returns an object which implements the genericIEnumerable(Of T) interface, which serves as the data source
for LINQ to DataSet queries.
In the query, you specify exactly the information that you want to retrieve from the data source. A query can also specify
how that information should be sorted, grouped, and shaped before it is returned. In LINQ, a query is stored in a variable. If
the query is designed to return a sequence of values, the query variable itself must be aenumerable type. This query variable
takes no action and returns no data; it only stores the query information. After you create a query you must execute that
query to retrieve any data.
In a query that returns a sequence of values, the query variable itself never holds the query results and only stores the query
commands. Execution of the query is deferred until the query variable is iterated over in a foreach or For Each loop. This is
called deferred execution; that is, query execution occurs some time after the query is constructed. This means that you can
execute a query as often as you want to. This is useful when, for example, you have a database that is being updated by other
applications. In your application, you can create a query to retrieve the latest information and repeatedly execute the query,
returning the updated information every time.
In contrast to deferred queries, which return a sequence of values, queries that return a singleton value are executed
immediately. Some examples of singleton queries are Count, Max, Average, and First. These execute immediately because
the query results are required to calculate the singleton result. For example, in order to find the average of the query results
the query must be executed so that the averaging function has input data to work with. You can also use the ToList(Of
TSource) or ToArray(Of TSource) methods on a query to force immediate execution of a query that does not produce a
singleton value. These techniques to force immediate execution can be useful when you want to cache the results of a query.
For more information about deferred and immediate query execution, see 6cc9af04-950a-4cc3-83d4-2aeb4abe4de9.
Queries
LINQ to DataSet queries can be formulated intwo different syntaxes: query expression syntax and method-based query
syntax.
Query Expression Syntax
Query expressions are a declarative query syntax. This syntax enables a developer to write queries in C# or Visual Basic
in a format similar to SQL. By using query expression syntax, you can perform even complex filtering, ordering, and
grouping operations on data sources with minimal code. For more information, see LINQ Query Expressions (C#
Programming Guide) and Basic Query Operations (Visual Basic).
.NET Framework (current version)
Queries in LINQ to DataSet
https://msdn.microsoft.com/en-us/library/bb552415(d=printer,v=vs.110).aspx
1 of 4 03.09.2016 0:40